目次
様々なフォームのカスタムフィールを作成
今回ご紹介するカスタムフィールドでのフォームは、カスタム投稿タイプでの作成になります。
下記コードを「functions.php」に、まるっとコピペしてカスタム投稿タイプを作成して下さい。
add_action('init', 'create_post_type');
function create_post_type()
{
//投稿時に使用できる投稿用のパーツを指定
$supports = array(
'title', //タイトルフォーム
'editor', //エディター(内容の編集)
'thumbnail', //アイキャッチ画像
'author', //投稿者
'excerpt', //抜粋
'revisions', //リビジョンを保存
);
register_post_type(
'sample',
[ // 投稿タイプ名の定義
'labels' => [
'name' => 'サンプル投稿', // 管理画面上で表示する投稿タイプ名
],
'public' => true, // カスタム投稿タイプの表示(trueにする)
'has_archive' => true, // カスタム投稿一覧(true:表示/false:非表示)
'menu_position' => 5, // 管理画面上での表示位置
'show_in_rest' => false, // true:「Gutenberg」/ false:「ClassicEditor」
'supports' => $supports
]
);
}
カスタム投稿タイプが完成したら、様々なフォームの紹介をしていきます。
※コールバック関数は、全て「insert_custom_fields」で記述しています。
まるっとコピペOKですので、テーマ開発者の方のお役に立てればと思います。
それでは、ご覧ください。
あわせて読みたい

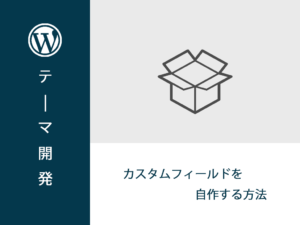
カスタムフィールドを自作する方法【プラグインなし】
【カスタムフィールドとは】 カスタムフィールドとは、「タイトル」や「本文」以外に、任意で自作フォームを作成できる機能のことをいいます。 【カスタムフィールドを…
テキストボックスをカスタムフィールドに作成
///////////////////まるっとコピペOK///////////////////
<?php
function insert_custom_fields()
{
global $post;
$sample = get_post_meta($post->ID, 'sample', true); ?>
<form method="post" action="admin.php?page=site_settings">
<label for="sample">サンプルフォーム:</label>
<input id="sample" type="text" name="sample" value="<?php echo $sample ?>">
</form>
<?php
}
add_action('save_post', 'save_custom_fields');
function save_custom_fields($post_id)
{
if (isset($_POST['sample'])) {
update_post_meta($post_id, 'sample', $_POST['sample']);
}
}
<?php echo get_post_meta($post->ID, "sample", true); ?>
テキストエリアをカスタムフィールドに作成
///////////////////まるっとコピペOK///////////////////
<?php
function insert_custom_fields()
{
global $post;
$sample = get_post_meta($post->ID, 'sample', true);
; ?>
<form method="post" action="admin.php?page=site_settings">
<label for="sample">サンプルテキストエリア:</label>
<textarea name="sample" id="sample" cols="30" rows="10" value="<?php echo $sample; ?>"><?php echo $sample; ?></textarea>
</form>
<?php
}
function save_custom_fields($post_id)
{
if (isset($_POST['sample'])) {
update_post_meta($post_id, 'sample', $_POST['sample']);
}
}
add_action('save_post', 'save_custom_fields');
<?php echo get_post_meta($post->ID, "sample", true); ?>
チェックボックスをカスタムフィールドに作成
<?php
function insert_custom_fields()
{
global $post;
$sample = get_post_meta($post->ID, 'sample', true);
if (get_post_meta($post->ID, 'sample', true) == "is-on") {
$sample_check = "checked";
} ?>
<form method="post" action="admin.php?page=site_settings">
<label for="sample">サンプルチェックボックス:</label>
<input id="sample" type="checkbox" name="sample" value="is-on" <?php echo $sample_check; ?>>
</form>
<?php
}
function save_custom_fields($post_id)
{
if (isset($_POST['sample'])) {
update_post_meta($post_id, 'sample', $_POST['sample']);
} else {
delete_post_meta($post_id, 'sample');
}
}
add_action('save_post', 'save_custom_fields');
<?php
if (get_post_meta($post->ID, "sample", true)) {
echo 'チェックされています。';
} else {
echo 'チェックされていません。';
}
?>
ラジオボタンをカスタムフィールドに作成
<?php
function insert_custom_fields()
{
global $post;
$sample = get_post_meta($post->ID, 'sample', true);
$sample1_checked = ($sample == 'sample1') ? 'checked': '';
$sample2_checked = ($sample == 'sample2') ? 'checked': '';
$sample3_checked = ($sample == 'sample3') ? 'checked': ''; ?>
<input type="radio" name="sample" value="sample1" <?php echo $sample1_checked; ?>>サンプル1<br>
<input type="radio" name="sample" value="sample2" <?php echo $sample2_checked; ?>>サンプル2<br>
<input type="radio" name="sample" value="sample3" <?php echo $sample3_checked; ?>>サンプル3
<?php
}
function save_custom_fields($post_id)
{
if (isset($_POST['sample'])) {
update_post_meta($post_id, 'sample', $_POST['sample']);
} else {
delete_post_meta($post_id, 'sample');
}
}
add_action('save_post', 'save_custom_fields');
<?php
if (get_post_meta($post->ID, "sample", true) == 'sample1') {
echo 'サンプル1が選択されています。';
} elseif (get_post_meta($post->ID, "sample", true) == 'sample2') {
echo 'サンプル2が選択されています。';
} else {
echo 'サンプル3が選択されています。';
}
?>
セレクトボックスをカスタムフィールドに作成
<?php
function insert_custom_fields()
{
global $post;
$sample = get_post_meta($post->ID, 'sample', true);
$sample1_selected = ($sample == 'sample1') ? 'selected': '';
$sample2_selected = ($sample == 'sample2') ? 'selected': '';
$sample3_selected = ($sample == 'sample3') ? 'selected': ''; ?>
<select id="sample" name="sample">
<option value="sample1" <?php echo $sample1_selected; ?>>サンプル1</option>
<option value="sample2" <?php echo $sample2_selected; ?>>サンプル2</option>
<option value="sample3" <?php echo $sample3_selected; ?>>サンプル3</option>
</select>
<?php
}
function save_custom_fields($post_id)
{
if (isset($_POST['sample'])) {
update_post_meta($post_id, 'sample', $_POST['sample']);
} else {
delete_post_meta($post_id, 'sample');
}
}
add_action('save_post', 'save_custom_fields');
<?php
if (get_post_meta($post->ID, "sample", true) == 'sample1') {
echo 'サンプル1が選択されています。';
} elseif (get_post_meta($post->ID, "sample", true) == 'sample2') {
echo 'サンプル2が選択されています。';
} else {
echo 'サンプル3が選択されています。';
}
?>